Web Technology 2074
Group A
Attempt any Two questions: (2x10=20)
AI is thinking...
1. Define HTTP. Explain the overall function of HTTP with example.
HTTP is a TCP/IP based communication protocol, that is used to deliver data (HTML files, image files, query results, etc.) on the World Wide Web. The default port is TCP 80, but other ports can be used as well. It provides a standardized way for computers to communicate with each other. HTTP specification specifies how clients' request data will be constructed and sent to the server, and how the servers respond to these requests.
There are three important features of HTTP:
- HTTP is connectionless: After a request is made, the client disconnects from the server and waits for a response. The server must re-establish the connection after it processes the request.
- HTTP is media independent: Any type of data can be sent by HTTP as long as both the client and server know how to handle the data content. How content is handled is determined by the MIME specification.
- HTTP is stateless: This is a direct result of HTTP's being connectionless. The server and client are aware of each other only during a request. Afterwards, each forgets the other. For this reason neither the client nor the browser can retain information between different requests across the web pages.
The following diagram shows a very basic architecture of a web application and depicts where HTTP sits:
The HTTP protocol is a request/response protocol based on the client/server based architecture where web browsers, robots and search engines, etc. act like HTTP clients, and the Web server acts as a server.
Client:
The HTTP client sends a request to the server in the form of a request method, URI, and protocol version, followed by a MIME-like message containing request modifiers, client information, and possible body content over a TCP/IP connection.
Server:
The HTTP server responds with a status line, including the message's protocol version and a success or error code, followed by a MIME-like message containing server information, entity meta information, and possible entity-body content.
AI is thinking...
2. Define XML schema. Compare it with DTD. Write a schema to describe "name" as a root element and "first_name","middle_name" and "last_name" as child elements.
XML schema is a language which is used for expressing constraint about XML documents. It is used to define the structure of an XML document. It is like DTD but provides more control on XML structure.
Comparison between DTD and XML schema
- XML schemas are written in XML while DTD are derived from SGML syntax.
- XML schemas define datatypes for elements and attributes while DTD doesn't support datatypes.
- XML schemas allow support for namespaces while DTD does not.
- XML schemas define number and order of child elements, while DTD does not.
- XML schemas can be manipulated on your own with XML DOM but it is not possible in case of DTD.
- using XML schema user need not to learn a new language but working with DTD is difficult for a user.
- XML schema provides secure data communication i.e. sender can describe the data in a way that receiver will understand, but in case of DTD data can be misunderstood by the receiver.
- XML schemas are extensible while DTD is not extensible.
XML schema to describe given elements
//name.xml
<?xml version="1.0" encoding="UTF-8"?>
<name xsi:schemalocation="name.xsd">
<first_name>Surya</first_name>
<middle_name>chandra</middle_name>
<last_name>Basnet</last_name>
</name>
// name.xsd
<?xml version="1.0" encoding="UTF-8"?>
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema">
<xs:element name="name">
<xs:complexType>
<xs:sequence>
<xs:element name="first_name" type="xs:string"/>
<xs:element name="middle_name" type="xs:string"/>
<xs:element name="last_name" type="xs:string"/>
</xs:sequence>
</xs:complexType>
</xs:element>
</xs:schema>
AI is thinking...
3. Construct a web page that takes name, address, and phone number from the user and stores this information in the database using server side script. Use JavaScript to validate form data for phone number.
//index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Form Registration</title>
</head>
<body>
<h2>Client Side Form Validation</h2>
<form name="signupForm" onsubmit="return validateForm();" method="POST" action="./formSubmit.php">
<div>
<label for="name">Please enter your name: </label>
<input type="text" name="name" id="name">
</div>
<div>
<label for="address">Please enter your address: </label>
<input type="text" name="address" id="address">
</div>
<div>
<label for="phoneNumber">Please enter your PhoneNumber: </label>
<input type="text" name="phoneNumber" id="PhoneNumber">
</div>
<div>
<input type="submit" name="signup" value="Signup">
</div>
</form>
<script>
function validateForm() {
if (document.signupForm.name.value == "") {
alert("Please provide your name!");
document.signupForm.name.focus();
return false;
}
else if (document.signupForm.address.value == "") {
alert("Please provide your address!");
document.signupForm.address.focus();
return false;
}
else if (document.signupForm.phoneNumber.value == "") {
alert("Please provide your PhoneNumber!");
document.signupForm.phoneNumber.focus();
return false;
}
else if (document.signupForm.phoneNumber.value.length < 10) {
alert('phoneNumber must be at least 10 digit long');
document.signupForm.name.focus();
return false;
}
return true;
}
</script>
</body>
</html>
//formSubmit.php
<?php
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "webtech";
$tablename = "users";
if($_SERVER["REQUEST_METHOD"] == "POST"){
$name = $_POST['name'];
$address = $_POST['address'];
$phoneNumber = $_POST['phoneNumber'];
//connect to database
$conn = mysqli_connect($servername, $username, $password) or die(mysql_error()); //Connect to server
mysqli_select_db($conn, $dbname) or die("Cannot connect to database"); //Connect to database
// Insert the values into database
mysqli_query($conn, "INSERT INTO ".$tablename."(name, address, phoneNumber) VALUES ('$name','$address', '$phoneNumber')");
Print '<script>alert("Congrats! Your Submission is Successfully Registered!");</script>';
?>
AI is thinking...
Group B
Attempt any Eight questions: (8x5=40)
AI is thinking...
4. What is HTML? Differentiate between "get" and "post" methods used to send form data.
HTML (Hyper Text Markup Language) is the standard markup language for creating Web pages. It describes the structure of a Web page.
Difference between GET and POST method
GET method | POST method |
GET is used to request data from a specified resource. | POST is used to send data to a server to create/update a resource. |
Data is visible to everyone in the URL. | Data is not displayed in the URL. |
Parameters remain in browser history. | Parameters are not saved in browser history. |
GET request can be cached. | POST requests are never cached. |
GET request can be bookmarked. | POST requests cannot be bookmarked. |
GET is less secure compared to POST because data is visible in the URL. | POST is a litter safer than GET because the parameters are not saved in browser history. |
GET request may have length restrictions. | POST requests have no restriction on data length. |
GET method should not be used when sending passwords or sensitive information. | POST method used when sending password or other sensitive information. |
Only ASCII characters are allowed. | No restrictions. Binary data is also allowed. |
AI is thinking...
5. What is CSS? How can you use an internal CSS? Explain.
CSS is a language that describes the style of an HTML document. It describes how HTML elements should be displayed.
Internal CSS
The internal style sheet is used to add a unique style for a single document. It is defined in <head> section of the HTML page inside the <style> tag.
E.g.
<!DOCTYPE html>
<html>
<head>
<style>
body {
background-color: linen;
}
h1 {
color: red;
margin-left: 80px;
}
</style>
</head>
<body>
<h1>The internal style sheet is applied on this heading.</h1>
<p>This paragraph will not be affected.</p>
</body>
</html>
AI is thinking...
6. How can you define event handler? Write a JavaScript to demonstrate event handling.
Event handlers are JavaScript code that are not added inside the <script> tags, but rather, inside the html tags, that execute JavaScript when something happens, such as pressing a button, moving your mouse over a link, submitting a form etc. The basic syntax of these event handlers is:
name_of_handler="JavaScript code here"
For example:
<a href="http://google.com" onClick="alert('hello!')">Google</a>
When the above link is clicked, the user will first see an alert message before being taken to Google.
AI is thinking...
7. What are the advantages of using XML? Explain the rules for writing XML.
Advantages of using XML
- Simplicity: Information coded in XML is easy to read and understand, plus it can be processed easily by computers.
- Openness: XML is a W3C standard, endorsed by software industry market leaders.
- Extensibility: There is no fixed set of tags. New tags can be created as they are needed.
- Self-description: XML documents can be stored without [schemas] because they contain meta data; any XML tag can possess an unlimited number of attributes such as author or version.
- Contains machine-readable context information: Tags, attributes and element structure provide context information ... opening up new possibilities for highly efficient search engines, intelligent data mining, agents, etc.
- Separates content from presentation: XML tags describe meaning not presentation. The look and feel of an XML document can be controlled by XSL stylesheets, allowing the look of a document (or of a complete Web site) to be changed without touching the content of the document. Multiple views or presentations of the same content are easily rendered.
- Supports multilingual documents and Unicode: This is important for the internationalization of applications.
- Facilitates the comparison and aggregation of data: The tree structure of XML documents allows documents to be compared and aggregated efficiently element by element.
- Can embed multiple data types: XML documents can contain any possible data type — from multimedia data (image, sound, video) to active components (Java applets, ActiveX).
- Can embed existing data: Mapping existing data structures like file systems or relational databases to XML is simple....
- Provides a “one-server view” for distributed data: XML documents can consist of nested elements that are distributed over multiple remote servers. XML is currently the most sophisticated format for distributed data — the World Wide Web can be seen as one huge XML database.
Rules for writing XML
1. All XML Elements Must Have a Closing Tag. In XML, it is illegal to omit the closing tag. All elements must have a closing tag:
E.g. Correct: <p>This is a paragraph.</p>
Incorrect: <p>This is a paragraph.
2. XML tags are case sensitive. The tag <Letter> is different from the tag <letter>. Opening and closing tags must be written with the same case:
E.g. Incorrect: <Message>This is incorrect</message>
Correct: <message>This is correct</message>
3. In XML, all elements must be properly nested within each other:
E.g. Incorrect: <b><i>This text is bold and italic</b></i>
Correct: <b><i>This text is bold and italic</i></b>
4. XML Documents Must Have a Root Element. XML documents must contain one element that is the parent of all other elements. This element is called the root element.
<root>
<child>
<subchild>.....</subchild>
</child>
</root>
5. XML Attribute Values Must be Quoted.
<note date="06/01/2012">
<to>Tulsi</to>
<from>Giri</from>
</note>
6. Comments in XML.
The syntax for writing comments in XML is similar to that of HTML.
<!-- This is a comment -->
7. White-space is preserved in XML.
8. Avoid HTML tags.
AI is thinking...
8. Discuss the use of Session with suitable example.
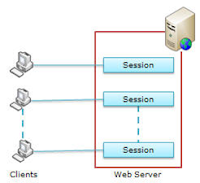
- Carrying information as a client travels between pages.
- One page data can be stored in session variable and that data can be accessed from other pages.
- Session can help to uniquely identify each client from another
- Session is mostly used in ecommerce sites where there is shopping cart system.
- It helps maintain user state and data all over the application.
- It is easy to implement and we can store any kind of object.
- Stores client data separately.
- Session is secure and transparent from the user.
// Start the session
session_start();
?>
<!DOCTYPE html>
<html>
<body>
<?php
// Set session variables
$_SESSION["favcolor"] = "green";
$_SESSION["favanimal"] = "cat";
echo "Session variables are set.";
?>
</body>
</html>
GET PHP session variables values
session_start();
?>
<!DOCTYPE html>
<html>
<body>
<?php
// Echo session variables that were set on previous example
echo "Favorite color is " . $_SESSION["favcolor"] . ".<br>";
echo "Favorite animal is " . $_SESSION["favanimal"] . ".";
?>
</body>
</html>
AI is thinking...
9. Why do we need XSLT? Explain XSL<xsl:if> Element.
XSLT (Extensible Stylesheet Language Transformations) provides the ability to transform XML data from one format to another automatically. It is the recommended style sheet language for XML.
XSLT is far more sophisticated than CSS. With XSLT we can add/remove elements and attributes to or from the output file. We can also rearrange and sort elements, perform tests and make decisions about which elements to hide and display, and a lot more.
XSLT uses XPath to find information in an XML document.
<xsl:if> Element
The XSLT <xsl:if> element is used to specify a conditional test against the content of the XML file.
Syntax:
<xsl:if test="expression">
...some output if the expression is true...
</xsl:if>
E.g.
<?xml version="1.0" encoding="UTF-8"?>
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="/">
<html>
<body>
<h2>My CD Collection</h2>
<table border="1">
<tr bgcolor="#9acd32">
<th>Title</th>
<th>Artist</th>
<th>Price</th>
</tr>
<xsl:for-each select="catalog/cd">
<xsl:if test="price > 10">
<tr>
<td><xsl:value-of select="title"/></td>
<td><xsl:value-of select="artist"/></td>
<td><xsl:value-of select="price"/></td>
</tr>
</xsl:if>
</xsl:for-each>
</table>
</body>
</html>
</xsl:template>
</xsl:stylesheet>
The code above will only output the title and artist elements of the CDs that has a price that is higher than 10.
AI is thinking...
10. What is web client? Discuss its role on the internet.
A web client is an application program or web browser that communicates with web server using Hypertext transfer protocol. Web client acts as an interface between server and clients, through which messages are sent to web server. It collects the processed data from the web server. E.g. Google chrome, Internet explorer, opera, firefox, safari.
Roles of web client:
- It acts as an interface between server and client and displays web document to the client.
- Web client requests the server for the webpages and resources.
- It sends HTTP requests and get HTTP response.
- It stores the cookies for different websites.
- It helps to bookmark the websites.
AI is thinking...
11. Write a server-side code to insert data in the database.
PHP code to insert data in the database
The INSERT INTO statement is used to add new records to a MySQL table:
INSERT INTO table_name (column1, column2, column3,...)
VALUES (value1, value2, value3,...)
The following examples add a new record to the "Student" table:
<?php
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "myDB";
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$sql = "INSERT INTO Student (firstname, lastname, email)
VALUES ('Cristiano', 'Ronaldo', 'cr7@gmail.com')";
if ($conn->query($sql) === TRUE) {
echo "New record created successfully";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
$conn->close();
?>
AI is thinking...
12. Discuss about authentication by IP addressing and domain.
AI is thinking...
13. Write short notes on:
a) POP
b) XPath
a) POP
POP (Post Office Protocol) is also called as POP3 protocol. This is a protocol used by a mail server in conjunction with SMTP to receive and holds mails for hosts.
POP3 mail server receives e-mails and filters them into the appropriate user folders. When a user connects to the mail server to retrieve his mail, the messages are downloaded from mail server to the user's hard disk.
b) XPath
XPath is an official recommendation of the World Wide Web Consortium (W3C). It defines a language to find information in an XML file. It is used to traverse elements and attributes of an XML document. XPath provides various types of expressions which can be used to enquire relevant information from the XML document.
- Structure Definitions − XPath defines the parts of an XML document like element, attribute, text, namespace, processing-instruction, comment, and document nodes
- Path Expressions − XPath provides powerful path expressions select nodes or list of nodes in XML documents.
- Standard Functions − XPath provides a rich library of standard functions for manipulation of string values, numeric values, date and time comparison, node and QName manipulation, sequence manipulation, Boolean values etc.
- Major part of XSLT − XPath is one of the major elements in XSLT standard and is must have knowledge in order to work with XSLT documents.
- W3C recommendation − XPath is an official recommendation of World Wide Web Consortium (W3C).
AI is thinking...