Web Technologies 2071
Group A
Attempt any Two questions: (2x10=20)
1. Develop a website that checks validity of a user during the log-in process. Assume that the login data (username and password) are already stored in a database. Use client-side script to validate the user input during login process.
//index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Login Form</title>
</head>
<body>
<h2>Client Side Form Validation</h2>
<form name="loginForm" onsubmit="return validateForm();" method="POST" action="./formSubmit.php">
<div>
<label for="username">Please enter your username: </label>
<input type="text" name="username" id="username">
</div>
<div>
<label for="password">Please enter your password: </label>
<input type="password" name="password" id="password">
</div>
<div>
<input type="submit" name="login" value="login">
</div>
</form>
<script>
function validateForm() {
if (document.loginForm.username.value == "") {
alert("Please provide your usernamename!");
document.loginForm.username.focus();
return false;
}
else if (document.loginForm.password.value == "") {
alert("Please provide your password!");
document.loginForm.password.focus();
return false;
}
else if (document.loginForm.password.value.length < 8) {
alert('Password must be at least 8 digit long');
document.loginForm.password.focus();
return false;
}
return true;
}
</script>
</body>
</html>
//formSubmit.php
<?php
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "webtech";
$tablename = "users";
if($_SERVER["REQUEST_METHOD"] == "POST"){
$username = $_POST['username'];
$password = $_POST['password'];
//connect to database
$conn = mysqli_connect($servername, $username, $password) or die(mysql_error()); //Connect to server
mysqli_select_db($conn, $dbname) or die("Cannot connect to database"); //Connect to database
// Insert the values into database
mysqli_query($conn, "INSERT INTO ".$tablename."(username, password) VALUES ('$username','$password')");
Print '<script>alert("Congrats! Your Submission is Successfully Registered!");</script>';
?>
2. What is FTP? Discuss its functions in detail.
File transfer protocol (FTP) is a set of rules that computers follow for the transferring of files from one system to another over the internet. It is mainly used for transferring the web page files from their creator to the computer that acts as a server for other computers on the internet. It is also used for downloading the files to computer from other servers.
When a user wishes to engage in File transfer, FTP sets up a TCP connection to the target system for the exchange of control messages. These allow used ID and password to be transmitted and allow the user to specify the file and file action desired. Once file transfer is approved, a second TCP connection is set up for data transfer. The file is transferred over the data connection, without the overhead of headers, or control information at the application level. When the transfer is complete, the control connection is used to signal the completion and to accept new file transfer commands.
Fig: Basic model of FTP
FTP can be run in active or passive mode, which determines how the data connection is established. In active mode, the server initiates the data connection with the client after the client has established a connection on the command channel. In passive mode it is the other way round, the client initiates the data connection with the server.
FTP includes three different transmission modes: stream, block and compressed. In stream mode, data is sent as a continuous sequence of bytes to TCP; in block mode, data is formatted into blocks with headers and then passes it on to TCP; and in compressed mode, bytes are compacted using run-length encoding. Stream mode is the one most commonly used.
3. Discuss the benefits of using XML. Differentiate XML schema with DTD. Write an XML DTD to describe “person” as an element and “Name, address, phone-no, and Age” as its attributes.
XML (Extensible Markup Language) is a markup language that defines a set of rules for encoding documents in a format that is both human readable and machine readable. XML is designed to store and transport data.
Benefits of using XML
- Simplicity: Information coded in XML is easy to read and understand, plus it can be processed easily by computers.
- Openness: XML is a W3C standard, endorsed by software industry market leaders.
- Extensibility: There is no fixed set of tags. New tags can be created as they are needed.
- Self-description: XML documents can be stored without [schemas] because they contain meta data; any XML tag can possess an unlimited number of attributes such as author or version.
- Contains machine-readable context information: Tags, attributes and element structure provide context information ... opening up new possibilities for highly efficient search engines, intelligent data mining, agents, etc.
- Separates content from presentation: XML tags describe meaning not presentation. The look and feel of an XML document can be controlled by XSL stylesheets, allowing the look of a document (or of a complete Web site) to be changed without touching the content of the document. Multiple views or presentations of the same content are easily rendered.
- Supports multilingual documents and Unicode: This is important for the internationalization of applications.
- Facilitates the comparison and aggregation of data: The tree structure of XML documents allows documents to be compared and aggregated efficiently element by element.
- Can embed multiple data types: XML documents can contain any possible data type — from multimedia data (image, sound, video) to active components (Java applets, ActiveX).
- Can embed existing data: Mapping existing data structures like file systems or relational databases to XML is simple....
- Provides a “one-server view” for distributed data: XML documents can consist of nested elements that are distributed over multiple remote servers. XML is currently the most sophisticated format for distributed data — the World Wide Web can be seen as one huge XML database.
Difference between XML schema and DTD
- XML schemas are written in XML while DTD are derived from SGML syntax.
- XML schemas define datatypes for elements and attributes while DTD doesn't support datatypes.
- XML schemas allow support for namespaces while DTD does not.
- XML schemas define number and order of child elements, while DTD does not.
- XML schemas can be manipulated on your own with XML DOM but it is not possible in case of DTD.
- using XML schema user need not to learn a new language but working with DTD is difficult for a user.
- XML schema provides secure data communication i.e. sender can describe the data in a way that receiver will understand, but in case of DTD data can be misunderstood by the receiver.
- XML schemas are extensible while DTD is not extensible.
XML DTD to describe given element and attributes
<?xml version="1.0" encoding="utf-8" ?>
<!DOCTYPE person
[
<!ELEMENT person EMPTY>
<!ATTLIST person Name CDATA #REQUIRED >
<!ATTLIST person address CDATA #REQUIRED >
<!ATTLIST person phone-no CDATA #REQUIRED >
<!ATTLIST person Age CDATA #REQUIRED >
]>
<person Name="Jayanta" address="Ramechhap" phone-no="9843000000" Age="20"></person>
Group B
Attempt any Eight questions: (8x5=40)
4. Discuss internet as a client/server technology.
Client-server is a computer model that separates client and server, and usually interlinked using a computer network. Each instance of a client can send data requests to one of the servers online and expect a response. In turn, some of the available servers can accept these requests, process them and return the result to the client. Often clients and servers communicate through a computer network with separate hardware, but the client and server can reside on the same system. The machine is a host server that is running one or more server programs that share their resources with clients. A client does not share its resources, but requests content from a server or service function. Clients, therefore, initiate communication sessions with the servers that wait for incoming requests.
Internet is a client/server technology because most services that are accessed via the Internet work on the client/server model. In that, we have a server which provides a service, while clients connect to the server to make requests regarding the service. A web site is an example: a web server holds the contents of the web site. Our web browser is a client, which we can use to connect to web servers to ask them for information, or to perform services on our behalf.
5. Discuss the structure of HTML document with suitable example.
HTML (Hyper Text Markup Language) is the standard markup language for creating Web pages. It describes the structure of a Web page.
Structure of HTML document
Every HTML document consists of four basic structure elements: html, head, title, and body. Each of these is explored in detail below:
1. Html Element
- Start tag:
<html>
- First tag in the document which declares you are writing an HTML element. - End tag:
</html>
- Last tag in the document.
2. Head Element
- Start tag:
<head>
- Second tag, follows the<html>
tag, and starts the head section, which describes the document. - End tag:
</head>
- Fifth tag, follows the</title>
tag and closes the head section.
3. Title Element
- Start tag:
<title>
- Third tag, follows the<head>
tag, and contains the title you want for the document. This information will be displayed in the title bar at the top of the browser window. - End tag:
</title>
- Fourth tag, immediately follows, without any spaces, the title you want for the document and precedes the</head>
tag.
4. Body Element
- Start tag:
<body>
- Sixth tag, follows the</head>
tag to denote starting the content of the document. - End tag:
</body>
- Next to last tag in the document, follows the end of the document content and precedes the</html>
tag.
E.g.
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<h1>My First Heading</h1>
<p>My first paragraph.</p>
</body>
</html>
6. Write an HTML code to display the following:
<!DOCTYPE html>
<html>
<head>
<title>Login Form</title>
<style>
#form1{border: 1px solid; padding: 20px; width: 300px;}
</style>
</head>
<body>
<form id="form1">
<label>Username:</label>
<input id="txtUserName" type="text" /><br /><br />
<label>Password:</label>
<input id="txtPassword" type="text" /><br /><br />
<input type="button" value="Login" />
</form>
</body>
</html>
7. How can you insert internal style sheet in your HTML document? Discuss with example.
The internal style sheet is used to add a unique style for a single document. It is defined in <head> section of the HTML page inside the <style> tag.
E.g.
<!DOCTYPE html>
<html>
<head>
<style>
body {
background-color: linen;
}
h1 {
color: red;
margin-left: 80px;
}
</style>
</head>
<body>
<h1>The internal style sheet is applied on this heading.</h1>
<p>This paragraph will not be affected.</p>
</body>
</html>
8. Write an example of client side script to validate a form.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Form Registration</title>
</head>
<body>
<h2>Client Side Form Validation</h2>
<form name="signupForm" onsubmit="return validateForm();" method="POST" action="">
<div>
<label for="username">Please enter your username: </label>
<input type="text" name="username" id="username">
</div>
<div>
<label for="password">Please enter your password: </label>
<input type="password" name="password" id="password">
</div>
<div>
<label for="email">Please enter your email: </label>
<input type="email" name="email" id="email">
</div>
<div>
<input type="submit" name="signup" value="Signup">
</div>
</form>
<script>
function validateForm() {
if (document.signupForm.username.value == "") {
alert("Please provide your usernamename!");
document.signupForm.username.focus();
return false;
}
else if (document.signupForm.password.value == "") {
alert("Please provide your password!");
document.signupForm.password.focus();
return false;
}
else if (document.signupForm.password.value.length < 8) {
alert('Password must be at least 8 digit long');
document.signupForm.password.focus();
return false;
}
else if (document.signupForm.email.value == "") {
alert("Please provide your Email!");
document.signupForm.email.focus();
return false;
}
return true;
}
</script>
</body>
</html>
9. What is HTTP?
HTTP is a TCP/IP based communication protocol, that is used to deliver data (HTML files, image files, query results, etc.) on the World Wide Web. The default port is TCP 80, but other ports can be used as well. It provides a standardized way for computers to communicate with each other. HTTP specification specifies how clients' request data will be constructed and sent to the server, and how the servers respond to these requests.
There are three important features of HTTP:
- HTTP is connectionless: After a request is made, the client disconnects from the server and waits for a response. The server must re-establish the connection after it processes the request.
- HTTP is media independent: Any type of data can be sent by HTTP as long as both the client and server know how to handle the data content. How content is handled is determined by the MIME specification.
- HTTP is stateless: This is a direct result of HTTP's being connectionless. The server and client are aware of each other only during a request. Afterwards, each forgets the other. For this reason neither the client nor the browser can retain information between different requests across the web pages.
10. Discuss the rules of writing XML document.
The syntax rules of XML are very simple and logical. The rules are easy to learn, and easy to use.
Rules for writing XML document:
1. All XML Elements Must Have a Closing Tag. In XML, it is illegal to omit the closing tag. All elements must have a closing tag:
E.g. Correct: <p>This is a paragraph.</p>
Incorrect: <p>This is a paragraph.
2. XML tags are case sensitive. The tag <Letter> is different from the tag <letter>. Opening and closing tags must be written with the same case:
E.g. Incorrect: <Message>This is incorrect</message>
Correct: <message>This is correct</message>
3. In XML, all elements must be properly nested within each other:
E.g. Incorrect: <b><i>This text is bold and italic</b></i>
Correct: <b><i>This text is bold and italic</i></b>
4. XML Documents Must Have a Root Element. XML documents must contain one element that is the parent of all other elements. This element is called the root element.
<root>
<child>
<subchild>.....</subchild>
</child>
</root>
5. XML Attribute Values Must be Quoted.
<note date="06/01/2012">
<to>Tulsi</to>
<from>Giri</from>
</note>
6. Comments in XML.
The syntax for writing comments in XML is similar to that of HTML.
<!-- This is a comment -->
7. White-space is preserved in XML.
8. Avoid HTML tags.
11. Why do we need session? Explain with suitable example.
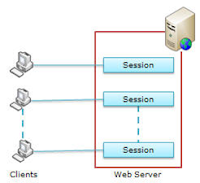
- Carrying information as a client travels between pages.
- One page data can be stored in session variable and that data can be accessed from other pages.
- Session can help to uniquely identify each client from another
- Session is mostly used in ecommerce sites where there is shopping cart system.
- It helps maintain user state and data all over the application.
- It is easy to implement and we can store any kind of object.
- Stores client data separately.
- Session is secure and transparent from the user.
// Start the session
session_start();
?>
<!DOCTYPE html>
<html>
<body>
<?php
// Set session variables
$_SESSION["favcolor"] = "green";
$_SESSION["favanimal"] = "cat";
echo "Session variables are set.";
?>
</body>
</html>
GET PHP session variables values
session_start();
?>
<!DOCTYPE html>
<html>
<body>
<?php
// Echo session variables that were set on previous example
echo "Favorite color is " . $_SESSION["favcolor"] . ".<br>";
echo "Favorite animal is " . $_SESSION["favanimal"] . ".";
?>
</body>
</html>
12. Discuss server side file handling with example.
File handling simply deal with creating, reading, deleting and editing files. Website can handle local files. It can read from, and write to, a text file on the disk. The code can run on any server with file system privileges—and also a local development machine
fopen()
function opens the file. The first parameter of fopen()
contains the name of the file to be opened and the second parameter specifies in which mode the file should be opened. E.g.PHP Read File - fread():
fread()
function reads from an open file. The first parameter of fread()
contains the name of the file to read from and the second parameter specifies the maximum number of bytes to read. The following PHP code reads the "webdictionary.txt" file to the end:PHP Close File - fclose():
fclose()
function is used to close an open file. E.g.PHP Write to File - fwrite():
fwrite()
function is used to write to a file. The first parameter of fwrite()
contains the name of the file to write to and the second parameter is the string to be written. E.g.13. Write short notes on:
a.
Tag libraries
b.
Anonymous access
a. Tag Libraries
In a Web application, a common design goal is to separate the display code from business logic. Java tag libraries are one solution to this problem. Tag libraries allow you to isolate business logic from the display code by creating a Tag class (which performs the business logic) and including an HTML-like tag in your JSP page. When the Web server encounters the tag within your JSP page, the Web server will call methods within the corresponding Java Tag class to produce the required HTML content.
A tag library defines a collection of custom actions. The tags can be used directly by developers in manually coding a JSP page, or automatically by Java development tools. A tag library must be portable between different JSP container implementations.
A custom tag library is made accessible to a JSP page through a taglib
directive of the following general form:
<%@ taglib uri="URI" prefix="prefix" %>
b. Anonymous access
Authentication is the process of determining the identity of a user based on the user’s credentials. The user’s credentials are usually in the form of user ID and password, which is checked against any credentials' store such as database. If the credentials provided by the user are valid, then the user is considered an authenticated user.