Web Technologies 2071-II
Group A
Attempt any Two questions: (2x10=20)
1. Develop a simple website that asks the users to register using username, password, and email address. Store these information’s in a database. Use client side script to validate the user input during registration.
//index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Form Registration</title>
</head>
<body>
<h2>Client Side Form Validation</h2>
<form name="signupForm" onsubmit="return validateForm();" method="POST" action="./formSubmit.php">
<div>
<label for="username">Please enter your username: </label>
<input type="text" name="username" id="username">
</div>
<div>
<label for="password">Please enter your password: </label>
<input type="password" name="password" id="password">
</div>
<div>
<label for="email">Please enter your email: </label>
<input type="email" name="email" id="email">
</div>
<div>
<input type="submit" name="signup" value="Signup">
</div>
</form>
<script>
function validateForm() {
if (document.signupForm.username.value == "") {
alert("Please provide your usernamename!");
document.signupForm.username.focus();
return false;
}
else if (document.signupForm.password.value == "") {
alert("Please provide your password!");
document.signupForm.password.focus();
return false;
}
else if (document.signupForm.password.value.length < 8) {
alert('Password must be at least 8 digit long');
document.signupForm.password.focus();
return false;
}
else if (document.signupForm.email.value == "") {
alert("Please provide your Email!");
document.signupForm.email.focus();
return false;
}
return true;
}
</script>
</body>
</html>
//formSubmit.php
<?php
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "webtech";
$tablename = "users";
if($_SERVER["REQUEST_METHOD"] == "POST"){
$username = $_POST['username'];
$password = $_POST['password'];
$email = $_POST['email'];
//connect to database
$conn = mysqli_connect($servername, $username, $password) or die(mysql_error()); //Connect to server
mysqli_select_db($conn, $dbname) or die("Cannot connect to database"); //Connect to database
// Insert the values into database
mysqli_query($conn, "INSERT INTO ".$tablename."(username, password, email) VALUES ('$username','$password', '$email')");
Print '<script>alert("Congrats! Your Submission is Successfully Registered!");</script>';
?>
2. What is XML? Discuss the structure of XML file with example. Write an XML schema to describe “address” as an element and “city, state, street and house-no” as its attributes.
XML (Extensible Markup Language) is a software- and hardware-independent tool for storing and transporting data. XML is a markup language that defines a set of rules for encoding documents in a format that is both human readable and machine readable. It has no predefined tags.
The major portions of an XML document include the following:
- The XML declaration: XML document can optionally have an XML declaration. It must be be the first statement of the XML document. The XML declaration is a processing instruction of the form <?xml........?>
- The document Type Declaration (DTD): It gives a name to the XML content, and provides a means to guarantee the document's validity either by including or specifying a link to a DTD. It is optional in XML.
- The element data: XML elements are either a matched pair of XML tags or single XML tags that are "self-closing". For e.g. A address element begins with <address> and ends with </address>. When elements do not come in pairs, the element name is suffixed by the forward slash. XML documents must contain one root element that is the parent of all other elements.
- The attribute data: It is additional information that can be communicated to XML processors They are name/value pairs contained within the start element. E.g. <price currency="USD">...</price>
- The character data or XML content: XML content can consists of any data, including binary data. It can contain any characters including any valid Unicode and International characters.
E.g. of XML
<?xml version="1.0" encoding="UTF-8"?>
<note>
<to> Sandhya </to>
<from> Barsha </from>
<heading> Reminder </heading>
<body> Don't forget me this weekend! </body>
</note>
XML schema for given elements and attributes
//Address.xml
<?xml version="1.0" encoding="utf-8" ?>
<Information xmlns:xsi="www.w3.org/2001/XMLSchema-Instance" xsi:noNameSpaceSchemaLocation="Address.xsd">
<Address City="Kathmandu" State="Bagmati" Street="Madhevsthan Road" House-No="110"></Address>
</Information>
//Address.xsd
<?xml version="1.0" encoding="utf-8"?>
<xs:schema xmlns:xsd="http://www.w3.or./2001.XMLSchema">
<xs:element name="Information">
<xs:complexType>
<xs:sequence>
<xs:element name="Address">
<xs:complexType>
<xs:attribute name="City" type="xsd:string" use="required">
<xs:attribute name="State" type="xsd:string" use="required">
<xs:attribute name="Street" type="xsd:string" use="required">
<xs:attribute name="House-No" type="xsd:string" use="required">
</xs:complexType>
</xs:element>
</xs:sequence>
</xs:complexType>
</xs:element>
</xs:schema>
3. What is HTTP? Discuss its function in detail.
HTTP is a TCP/IP based communication protocol, that is used to deliver data (HTML files, image files, query results, etc.) on the World Wide Web. The default port is TCP 80, but other ports can be used as well. It provides a standardized way for computers to communicate with each other. HTTP specification specifies how clients' request data will be constructed and sent to the server, and how the servers respond to these requests.
There are three important features of HTTP:
- HTTP is connectionless: After a request is made, the client disconnects from the server and waits for a response. The server must re-establish the connection after it processes the request.
- HTTP is media independent: Any type of data can be sent by HTTP as long as both the client and server know how to handle the data content. How content is handled is determined by the MIME specification.
- HTTP is stateless: This is a direct result of HTTP's being connectionless. The server and client are aware of each other only during a request. Afterwards, each forgets the other. For this reason neither the client nor the browser can retain information between different requests across the web pages.
The following diagram shows a very basic architecture of a web application and depicts where HTTP sits:
The HTTP protocol is a request/response protocol based on the client/server based architecture where web browsers, robots and search engines, etc. act like HTTP clients, and the Web server acts as a server.
Client:
The HTTP client sends a request to the server in the form of a request method, URI, and protocol version, followed by a MIME-like message containing request modifiers, client information, and possible body content over a TCP/IP connection.
Server:
The HTTP server responds with a status line, including the message's protocol version and a success or error code, followed by a MIME-like message containing server information, entity meta information, and possible entity-body content.
Group B
Attempt any Eight questions: (8x5=40)
4. What is client/server technology? Differentiate between web client and web server.
Client-server is a computer model that separates client and server, and usually interlinked using a computer network. Each instance of a client can send data requests to one of the servers online and expect a response. In turn, some of the available servers can accept these requests, process them and return the result to the client. Often clients and servers communicate through a computer network with separate hardware, but the client and server can reside on the same system. The machine is a host server that is running one or more server programs that share their resources with clients. A client does not share its resources, but requests content from a server or service function. Clients, therefore, initiate communication sessions with the servers that wait for incoming requests.
Difference between web client and web server
Web Client /web browsers | Web Server |
It is an application program that acts as an interface between server and client and displays web document to the client. | It is a program or the computer that provide services to other programs called client. |
Web client requests the server for the web pages and services. | Web server stores, process and deliver the web pages to web browsers. |
It sends HTTP requests and get HTTP response. | It gets HTTP requests and sends HTTP responses. |
The web browsers is installed on the client computer. | The web servers can be a remote machine placed at the other side of the network. |
Web browser stores the cookies for different websites. | Web servers provide an area to store and organize the pages of websites. |
It is a simple and less powerful machine. | It is powerful and expensive machine. |
It supports single user login at a time. | It supports multi-user login at a time. |
5. How can you draw a table in HTML? Discuss with suitable example.
Tables are defined with the <table> tag. A table is divided into rows (with the <tr> tag), and each row is divided into data cells (with the <td> tag). The letters td stands for "table data," which is the content of a data cell. A data cell can contain text, images, lists, paragraphs, forms, horizontal rules, tables, etc. Headings in a table are defined with <th> tag.
Table tags:
Table tags attributes:
E.g.
<!DOCTYPE html>
<html>
<head>
<title>Table</title>
</head>
<body>
<h1>The table element</h1>
<table border="1">
<tr>
<th>Month</th>
<th>Savings</th>
</tr>
<tr>
<td>January</td>
<td>$100</td>
</tr>
<tr>
<td>February</td>
<td>$80</td>
</tr>
</table>
</body>
</html>
6. Write and HTML code to display the following:
<!DOCTYPE html>
<html>
<head>
<title>Example</title>
</head>
<body>
<table border="1">
<tr>
<td><b>Example</b></td>
</tr>
<tr>
<td>(a+b)<sup>2</sup>=a<sup>2</sup>+ 2ab + b<sup>2</sup></td>
</tr>
<tr>
<td>Thank You</td>
</tr>
</table>
</body>
</html>
7. How can you insert external style sheets in your HTML document? Discuss with example.
External CSS contains separate CSS file which contains only style property with the help of tag attributes (For example class, id, heading, … etc). CSS property written in a separate file with .css extension and should be linked to the HTML document using link tag. This means that for each element, style can be set only once and that will be applied across web pages.
E.g.
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="style.css"/>
</head>
<body>
<div class = "main">
<div class ="CN">College Note</div>
<div id ="webtech">
Web tech solution.
</div>
</div>
</body>
</html>
//style.css
body {
background-color:powderblue;
}
.main {
text-align:center;
}
.CN {
color:#009900;
font-size:50px;
font-weight:bold;
}
#webtech {
font-style:bold;
font-size:20px;
}
8. Write a simple client side script to set and retrieve cookies.
JavaScript code to set and retrieve a cookie:
<!DOCTYPE html>
<html>
<head>
<title>Cookies</title>
</head>
<body>
<input type="button" value="setCookie" onclick="setCookie()">
<input type="button" value="getCookie" onclick="getCookie()">
<script>
function setCookie()
{
document.cookie="username=Jayanta";
}
function getCookie()
{
if(document.cookie.length!=0)
{
alert(document.cookie);
}
else
{
alert("Cookie not available");
}
}
</script>
</body>
</html>
9. What is FTP?
File transfer protocol (FTP) is a set of rules that computers follow for the transferring of files from one system to another over the internet. It is mainly used for transferring the web page files from their creator to the computer that acts as a server for other computers on the internet. It is also used for downloading the files to computer from other servers.
When a user wishes to engage in File transfer, FTP sets up a TCP connection to the target system for the exchange of control messages. These allow used ID and password to be transmitted and allow the user to specify the file and file action desired. Once file transfer is approved, a second TCP connection is set up for data transfer. The file is transferred over the data connection, without the overhead of headers, or control information at the application level. When the transfer is complete, the control connection is used to signal the completion and to accept new file transfer commands.
Fig: Basic model of FTP
10. Discuss public external declaration of DTD with example.
In external DTD elements are declared outside the XML file.
Public external DTDs are identified by the keyword PUBLIC and are intended for broad use. The "DTD_location" is used to find the public DTD if it cannot be located by the "DTD_name". Its syntax is:
<!DOCTYPE root_element PUBLIC "DTD_name" "DTD_location">.
where:
DTD_location: relative or absolute URL
DTD_name: follows the syntax:
"prefix//owner_of_the_DTD//description_of_the_DTD//ISO 639_language_identifier".
If any elements, attributes, or entities are used in the XML document that are referenced or defined in an external DTD, standalone="no" must be included in the XML declarationvalidity constraint.
For example,
<?xml version="1.0" standalone="no" ?>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.0 Transitional//EN"
"http://www.w3.org/TR/REC-html40/loose.dtd">
11. Define session. Explain its use with example.
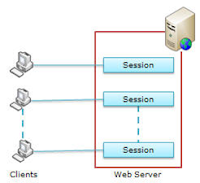
- Carrying information as a client travels between pages.
- One page data can be stored in session variable and that data can be accessed from other pages.
- Session can help to uniquely identify each client from another
- Session is mostly used in ecommerce sites where there is shopping cart system.
- It helps maintain user state and data all over the application.
- It is easy to implement and we can store any kind of object.
- Stores client data separately.
- Session is secure and transparent from the user.
// Start the session
session_start();
?>
<!DOCTYPE html>
<html>
<body>
<?php
// Set session variables
$_SESSION["favcolor"] = "green";
$_SESSION["favanimal"] = "cat";
echo "Session variables are set.";
?>
</body>
</html>
GET PHP session variables values
session_start();
?>
<!DOCTYPE html>
<html>
<body>
<?php
// Echo session variables that were set on previous example
echo "Favorite color is " . $_SESSION["favcolor"] . ".<br>";
echo "Favorite animal is " . $_SESSION["favanimal"] . ".";
?>
</body>
</html>
12. What are anti-overloaded techniques in web server?
At any time web servers can be overloaded because of:
- Too much legitimate web traffic
- Thousands or even millions of clients hitting the web site in a short interval of time (DDoS) Distributed Denial of Service attacks
- Computer worms
- Millions of infected browsers and/or web servers
- limited on large web sites with very few resources (bandwidth, etc.)
- Client requests are served more slowly and the number of connections increases so much that server limits are reached
- Web servers required urgent maintenance or upgrade
- Hardware Software failures
To partially overcome load limits and to prevent overload we can use techniques like:
- Managing network traffic by using: Firewalls, Block unwanted traffic
- HTTP traffic managers- redirect or rewrite requests having bad HTTP patterns
- Bandwidth management and traffic shaping
- Deploying web cache techniques
- Use different domains to serve different content (static and dynamic) by separate Web servers,
- Add more hardware resources
- Use many web servers
These technique to limit the load on websites are called anti-overload techniques in web server.
13. Write short notes on:
a. HTML DOM
b. Tag libraries
a. HTML DOM
The HTML DOM is an Object Model for HTML. It defines:
- HTML elements as objects
- Properties for all HTML elements
- Methods for all HTML elements
- Events for all HTML elements
When a web page is loaded, the browser creates a Document Object Model of the page. The HTML DOM model is constructed as a tree of Objects:
b. Tag libraries
In a Web application, a common design goal is to separate the display code from business logic. Java tag libraries are one solution to this problem. Tag libraries allow you to isolate business logic from the display code by creating a Tag class (which performs the business logic) and including an HTML-like tag in your JSP page. When the Web server encounters the tag within your JSP page, the Web server will call methods within the corresponding Java Tag class to produce the required HTML content.
A tag library defines a collection of custom actions. The tags can be used directly by developers in manually coding a JSP page, or automatically by Java development tools. A tag library must be portable between different JSP container implementations.
A custom tag library is made accessible to a JSP page through a taglib
directive of the following general form:
<%@ taglib uri="URI" prefix="prefix" %>