Web Technology 2076
Group A
Attempt any Two questions: (2x10=20)
AI is thinking...
1. Design a form containing text box for username, password field for password, radio button for gender and checkbox for hobbies. Write a program for client-side validation of the form for the username and password field as required fields, length of username should be 5, the radio button and checkbox should be checked.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Form validation</title>
<script>
function validateForm() {
var username = document.getElementById("username").value;
var password = document.getElementById("password").value;
var gender = document.getElementsByName("gender");
var hobbies = document.getElementsByName("hobbies");
if (username == "") {
alert('username is required');
return false;
}
else if (username.length !== 5) {
alert('username must be 5 characters long');
return false;
}
else if (password == "") {
alert('password is required');
return false;
}
else if (!(gender[0].checked || gender[1].checked || gender[2].checked)) {
alert("Please Select Your Gender");
return false;
}
else if (!(hobbies[0].checked || hobbies[1].checked || hobbies[2].checked || hobbies[3].checked)) {
alert("Please Select Atleast one of you hobby");
return false;
}
return true;
}
</script>
</head>
<body>
<h2>Client Side Form Validation Example</h2>
<form method="POST" action="#">
<div>
<label> Username: </label>
<input type="text" name="username" id="username" required>
</div><br>
<div>
<label> Password: </label>
<input type="password" name="password" id="password" required>
</div><br>
<div>
<label> Gender: </label>
<label><input type="radio" value="m" name="gender"> Male </label>
<label><input type="radio" value="f" name="gender"> Female </label>
<label><input type="radio" value="0" name="gender"> Others </label>
</div><br>
<div>
<label> Hobbies: </label>
<label><input type="checkbox" name="hobbies"> Reading </label>
<label><input type="checkbox" name="hobbies"> Writing </label>
<label><input type="checkbox" name="hobbies"> Playing </label>
<label><input type="checkbox" name="hobbies"> Music </label>
</div><br>
<button onclick="validateForm()">Submit</button>
</form>
</body>
</html>
AI is thinking...
2. What is the use of XSLT? Create a XML file with elements and elements having attributes. Write the equivalent XSLT of the XML for rendering as a HTML document in a browser.
XSLT (Extensible Stylesheet Language Transformations) is a language for transforming XML documents into other XML documents, or other formats such as HTML for web pages, plain text or XSL Formatting Objects, which may subsequently be converted to other formats, such as PDF, Postscript and PNG.
The original document is not changed; rather, a new document is created based on the content of an existing one. Typically, input documents are XML files, but anything from which the processor can build an XQuery and XPath Data Model can be used, such as relational database tables or geographical information system.
Creating Students.xml as:
<?xml version="1.0" encoding="UTF-8"?>
<?xml-stylesheet type="text/xsl "href="student.xsl" ?>
<student>
<s>
<name> Jayanta </name>
<branch> CS</branch>
<age>20</age>
<city> Kathmandu </city>
</s>
<s>
<name> Manoj </name>
<branch> IT </branch>
<age> 20</age>
<city> Pokhara </city>
</s>
<s>
<name> Sagar</name>
<branch> HM </branch>
<age> 23</age>
<city> Biratnagar </city>
</s>
</student>
Now, equivalent XSLT of the above XML for rendering as a HTML document in a browser is given below:
// student.xsl
<?xml version="1.0" encoding="UTF-8"?>
<xsl:stylesheet version="1.0"
xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="/">
<html>
<body>
<h1 align="center">Students' Basic Details</h1>
<table border="3" align="center" >
<tr>
<th>Name</th>
<th>Branch</th>
<th>Age</th>
<th>City</th>
</tr>
<xsl:for-each select="student/s">
<tr>
<td><xsl:value-of select="name"/></td>
<td><xsl:value-of select="branch"/></td>
<td><xsl:value-of select="age"/></td>
<td><xsl:value-of select="city"/></td>
</tr>
</xsl:for-each>
</table>
</body>
</html>
</xsl:template>
</xsl:stylesheet>
AI is thinking...
3. Prepare a form that should contain text box for name and email, selection list for country. Write a server side script to store data from the form into database. The script should contain connection to database and appropriate query into the database.
//index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Form Registration</title>
</head>
<body>
<form method="POST" action="formSubmit.php">
<div>
<label for="name">Please enter your name: </label>
<input type="text" name="name" id="name">
</div>
<div>
<label for="email">Please enter email: </label>
<input type="text" name="email" id="email">
</div>
<div>
<label for="country">Choose your Country: </label>
<select name="country>"
<option value="nepal">Nepal</option>
<option value="china">China</option>
<option value="india">India</option>
<option value="usa">USA</option>
</select>
</div>
<div>
<input type="submit" name="signup" value="Signup">
</div>
</form>
</body>
</html>
//formSubmit.php
<?php
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "webtech";
$tablename = "users";
if($_SERVER["REQUEST_METHOD"] == "POST"){
$name = $_POST['name'];
$email = $_POST['email'];
$country = $_POST['country'];
//connect to database
$conn = mysqli_connect($servername, $username, $password) or die(mysql_error()); //Connect to server
mysqli_select_db($conn, $dbname) or die("Cannot connect to database"); //Connect to database
// Insert the values into database
mysqli_query($conn, "INSERT INTO ".$tablename."(name, email, country) VALUES ('$name', '$email', '$country')");
Print '<script>alert("Congrats! Your Submission is Successfully Registered!");</script>';
?>
AI is thinking...
Group B
Attempt any Eight questions: (8x5=40)
AI is thinking...
4. How external style sheets can be inserted in a HTML document? Illustrate with example.
External CSS contains separate CSS file which contains only style property with the help of tag attributes (For example class, id, heading, … etc). CSS property written in a separate file with .css extension and should be linked to the HTML document using link tag. This means that for each element, style can be set only once and that will be applied across web pages.
E.g.
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="style.css"/>
</head>
<body>
<div class = "main">
<div class ="CN">College Note</div>
<div id ="webtech">
Web tech solution.
</div>
</div>
</body>
</html>
//style.css
body {
background-color:powderblue;
}
.main {
text-align:center;
}
.CN {
color:#009900;
font-size:50px;
font-weight:bold;
}
#webtech {
font-style:bold;
font-size:20px;
}
AI is thinking...
5. What do you mean by cookie? Write a Javascript code to handle cookie?
A cookie is a variable that is stored on the visitor's computer. Cookies let you store user information in web pages.
When a web server has sent a web page to a browser, the connection is shut down, and the server forgets everything about the user. Cookies were invented to solve the problem "how to remember information about the user".
- When a user visits a web page, his/her name can be stored in a cookie.
- Next time the user visits the page, the cookie "remembers" his/her name.
Cookies are saved in name-value pairs like:
username = Ronaldo
When a browser requests a web page from a server, cookies belonging to the page are added to the request. This way the server gets the necessary data to "remember" information about users.
JavaScript code to set and get a cookie:
<!DOCTYPE html>
<html>
<head>
</head>
<body>
<input type="button" value="setCookie" onclick="setCookie()">
<input type="button" value="getCookie" onclick="getCookie()">
<script>
function setCookie()
{
document.cookie="username=Jayanta";
}
function getCookie()
{
if(document.cookie.length!=0)
{
alert(document.cookie);
}
else
{
alert("Cookie not available");
}
}
</script>
</body>
</html>
AI is thinking...
6. Write HTML script for displaying table with following output.
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<table border="1">
<tr>
<th>Drinks</th>
<th>Snacks</th>
</tr>
<tr>
<td>
<ol>
<li>Coke</li>
<li>Sprite</li>
</ol>
</td>
<td>Momo</td>
</tr>
<tr>
<td>
<ul>
<li>Milk</li>
<li>Coffe</li>
</ul>
</td>
<td>Chowmin</td>
</tr>
</table>
</body>
</html>
AI is thinking...
7. What are the visibility and display properties of HTML elements? Explain with example.
AI is thinking...
8. Describe HTTP with its header fields.
HTTP is a TCP/IP based communication protocol, that is used to deliver data (HTML files, image files, query results, etc.) on the World Wide Web. The default port is TCP 80, but other ports can be used as well. It provides a standardized way for computers to communicate with each other. HTTP specification specifies how clients' request data will be constructed and sent to the server, and how the servers respond to these requests.
HTTP header fields provide required information about the request or response, or about the object sent in the message body. There are four types of HTTP message headers:
- General-header: These header fields have general applicability for both request and response messages.
- Request-header: These header fields have applicability only for request messages.
- Response-header: These header fields have applicability only for response messages.
- Entity-header: These header fields define meta information about the entity-body or, if no body is present, about the resource identified by the request.
All the above mentioned headers follow the same generic format and each of the header field consists of a name followed by a colon (:) and the field value as follows:
message-header = field-name ":" [ field-value ]
AI is thinking...
9. Discuss different types of client server architectures.
Client server architecture consists of two kinds of computers: clients and servers. Clients are the computers that that do not share any of its resources but requests data and other services from the server computers and server computers provide services to the client computers by responding to client computers requests.
Client/Sever architecture can be of different model based on the number of layers it holds. Some of them are;
1. 2-Tier Architecture
2-tier architecture is used to describe client/server systems where the client requests resources and the server responds directly to the request, using its own resources. This means that the server does not call on another application in order to provide part of the service. It runs the client processes separately from the server processes, usually on a different computer.
2. 3-Tier Architecture
In 3-tier architecture, there is an intermediary level, meaning the architecture is generally split up between:
– A client, i.e. the computer, which requests the resources, equipped with a user interface (usually a web browser) for presentation purposes
– The application server (also called middleware), whose task it is to provide the requested resources, but by calling on another server
– The data server, which provides the application server with the data it requires.
3. N-Tier Architecture (multi-tier)
N-tier architecture (with N more than 3) is really 3 tier architectures in which the middle tier is split up into new tiers. The application tier is broken down into separate parts. These parts are differs from system to system.
AI is thinking...
10. Consider a XML file containing element name city <city> </city>. Now write its equivalent XSD to limit the content of city to a set of acceptable values from "PKR", "KTM", "NPJ".
<?xml version="1.0" encoding="UTF-8" ?>
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema">
<xs:element name="city">
<xs:simpleType>
<xs:restriction base="xs:string">
<xs:enumeration value="PKR"/>
<xs:enumeration value="KTM"/>
<xs:enumeration value="NPJ"/>
</xs:restriction>
</xs:simpleType>
</xs:element>
</xs:schema>
AI is thinking...
11. How attributes and elements are defined in a DTD? Illustrate with an example.
DTD stands for Document Type Definition. It defines the legal building blocks of an XML document. It is used to define document structure with a list of legal elements and attributes. Its main purpose is to define the structure of an XML document. It contains a list of legal elements and define the structure with the help of them.
Elements in DTD
In a DTD, elements are declared with an ELEMENT declaration. In DTD, XML elements are declared with the following syntax:
<!ELEMENT element-name category>
or
<!ELEMENT element-name (element-content)>
E.g.
<?xml version="1.0"?>
<!DOCTYPE note [
<!ELEMENT note (to,from,heading,body)>
<!ELEMENT to (#PCDATA)>
<!ELEMENT from (#PCDATA)>
<!ELEMENT heading (#PCDATA)>
<!ELEMENT body (#PCDATA)>
]>
<note>
<to>Tove</to>
<from>Jani</from>
<heading>Reminder</heading>
<body>Don't forget me this weekend</body>
</note>
Attributes in DTD
In a DTD, attributes are declared with an ATTLIST declaration. An attribute declaration has the following syntax:
<!ATTLIST element-name attribute-name attribute-type attribute-value>
E.g.
DTD example:
<!ATTLIST employee id CDATA #REQUIRED>
XML example:
<employee id="001" />
AI is thinking...
12. How session handling is done in server side scripts?
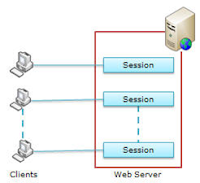
// Start the session
session_start();
?>
<!DOCTYPE html>
<html>
<body>
<?php
// Set session variables
$_SESSION["favcolor"] = "green";
$_SESSION["favanimal"] = "cat";
echo "Session variables are set.";
?>
</body>
</html>
GET PHP session variables values
session_start();
?>
<!DOCTYPE html>
<html>
<body>
<?php
// Echo session variables that were set on previous example
echo "Favorite color is " . $_SESSION["favcolor"] . ".<br>";
echo "Favorite animal is " . $_SESSION["favanimal"] . ".";
?>
</body>
</html>
AI is thinking...
13. Write short notes on:
a) XML Namespace
b) HTML DOM
a. XML Namespace
XML Namespace is a mechanism to avoid name conflicts by differentiating elements or attributes within an XML document that may have identical names, but different definitions.
Consider two XML fragment:
//1.xml
<table>
<tr>
<td>Apples</td>
<td>Bananas</td>
</tr>
</table>
//2.xml
<table>
<name>African Coffee Table</name>
<width>80</width>
<length>120</length>
</table>
If these XML fragments were added together, there would be a name conflict. Both contain a <table> element, but the elements have different content and meaning.
Name conflicts in XML can easily be avoided using a name prefix.
E.g.
<h:table>
<h:tr>
<h:td>Apples</h:td>
<h:td>Bananas</h:td>
</h:tr>
</h:table>
<f:table>
<f:name>African Coffee Table</f:name>
<f:width>80</f:width>
<f:length>120</f:length>
</f:table>
In this example, there will be no conflict because the two <table> elements have different names.
b. HTML DOM
The HTML DOM is an Object Model for HTML. It defines:
- HTML elements as objects
- Properties for all HTML elements
- Methods for all HTML elements
- Events for all HTML elements
When a web page is loaded, the browser creates a Document Object Model of the page. The HTML DOM model is constructed as a tree of Objects:
AI is thinking...